Robot Skill Language
The modeling relies on a language that we want to be unambiguous and that allows describing the capabilities of different robots. It is called robot-language. This language supports the modeling, the various analyses, and the generation of the code that is then embedded and deployed on different robots. It is important to notice that the runtime semantics of a skillset relies on three distinct elements: its model defined using robot-language; its functional definition (the skillset implementation) implemented by C++ code placed in specific locations (called hook), and its use by the decision layer which produces (so-called external) requests on the skillset.
Skillset
A skillset, as the name suggests, represents the different capabilities of a robot and the accessible data and resources it uses. Each skillset is composed of data, resources, events and skills.
1// comment
2skillset toto {
3 data { }
4 resource { }
5 event { }
6 skill { }
7}
Skillset Data
Each skillset has data that represents the data it owns and makes available to other elements of the architecture. This data is characterized by a name and a type, and may have a period. The data can be retrieved by three different means:
when one of the data is modified within the skillset, it is then automatically published;
by queries;
periodically if the period is specified in the robot-language model; in that case, the period is specified in seconds.
1data {
2 battery : Battery
3 position: GeoPoint period 1.0
4 home : GeoPoint
5}
Skillset Resource
As the name suggests, resource are intended to represent resources, whether they are hardware (e.g. sensors) or software/conceptual (e.g. authority). Using the robot-language specification, a resource is a state-machine defined by a name, an initial state, and a set of transitions between states. The resource is an important concept of the execution model of the skillset architecture because it allows specifying the conditions of operation, and the impact of the robots’ actions on the system. Consequently, and we will detail it later, resources are used in the preconditions, invariant, and different effects of the robots’ skills. The state of the different resources is both automatically published at each modification of the internal state of the skillset and also on request.
1 resource {
2 authority {
3 state { Free Pilot Software }
4 initial Pilot
5 transition {
6 Free -> Software
7 Free -> Pilot
8 Software -> Free
9 Software -> Pilot
10 Pilot -> Free
11 }
12 }
13 flight_status {
14 state { NotReady OnGround InAir }
15 initial NotReady
16 transition all
17 }
18 motion {
19 state { Available Used }
20 initial Available
21 transition all
22 }
23 battery {
24 state { Good Low Critical }
25 initial Good
26 transition {
27 Good -> Low
28 Good -> Critical
29 Low -> Critical
30 }
31 }
Resources are also used in guards and effects. A resource guard guard is a logic formula on the state of the resources. The guard is used in the events, the preconditions, and the invariants of the skills. A resource effect aims to change the state of a specific resource. It is defined by the name of the resource and its next state, and is called an arc.
Skillset Event
The purpose of events is to enable state changes on one or more resources, from outside the skillset manager. An event is defined by its name, a guard (optional), the effects (optional), and a hook. The guard and the effect are defined in the robot-language specification. The event hook is a piece of code defined by the developer of the skillset. The result of an event can be success, guard_failure if the guard is not satisfied when the event is called, or effets_failure if the effects cannot be applied.
1 event take_authority {
2 guard authority != Pilot
3 effect authority -> Pilot
4 }
Skillset Skill
The robot-language allows skills to be defined using the following elements:
inputs are used in the functional algorithm of the skill. Each input is defined as a data and thus has a name and a type.
outputs are data produced at the end of the execution of the skill.
preconditions are used to check if the skill can start its execution properly. Each skill can have zero, one, or more preconditions.
start is used to define some effects on the resources of the skillset before starting.
invariant is used to specify mandatory properties to the execution of the skill. If at least one invariant is violated, then the skill is stopped.
progress is used to monitor the execution of the skill. It produces monitoring data periodically during the execution of the skill.
interrupt is used to specify the effects applied after the skill is interrupted. Any skill can be interrupted.
success is used to specify the different execution success states of the skill and their corresponding effects on the resources after its completion.
failure is used to specify the different execution failure states of the skill and their corresponding effects on the resources after its completion.
postconditions are used to specify the states of the resources at the end of the execution of the skill. Contrary to the effects, postconditions is a resource formula and the real effect is done by the functional layer. Invariants, success, and failure states can have postconditions.
The internal behavior of a skill follows the state-machine depicted in the following figure. When a skill is called then the first step is to check each precondition, if one fails then the skill stops and returns an error, if all the preconditions are satisfied then the skill continues its internal state-machine to the validation step. The validation step consists in running the validation hook. If the validation hook fails, then the request is terminated, otherwise, the skill continues its internal state-machine and the effects specified in the start block proceed by checking if all its effects are possible. If at least one effect is not possible, then the skill returns a “start error”. If all are possible, then they are applied. Once the start is executed without error, the skill is running and then the invariant loop function is called to check all the invariants of the skillset. The invariant loop can lead other skills to terminate with an invariant failure, and the effects of those invariant failures can also imply other invariant failures, and so on. The invariant loop terminates because a stopped skill cannot re-enter in the running state. While running, the skill can finish its execution with an invariant failure or terminate its execution: by being interrupted or can succeed or fail. The interrupt is an external request as the start of the skill is. The success or the failure of a skill is an internal call from the functional part of the skillset that also specifies the success (resp. failure) state.
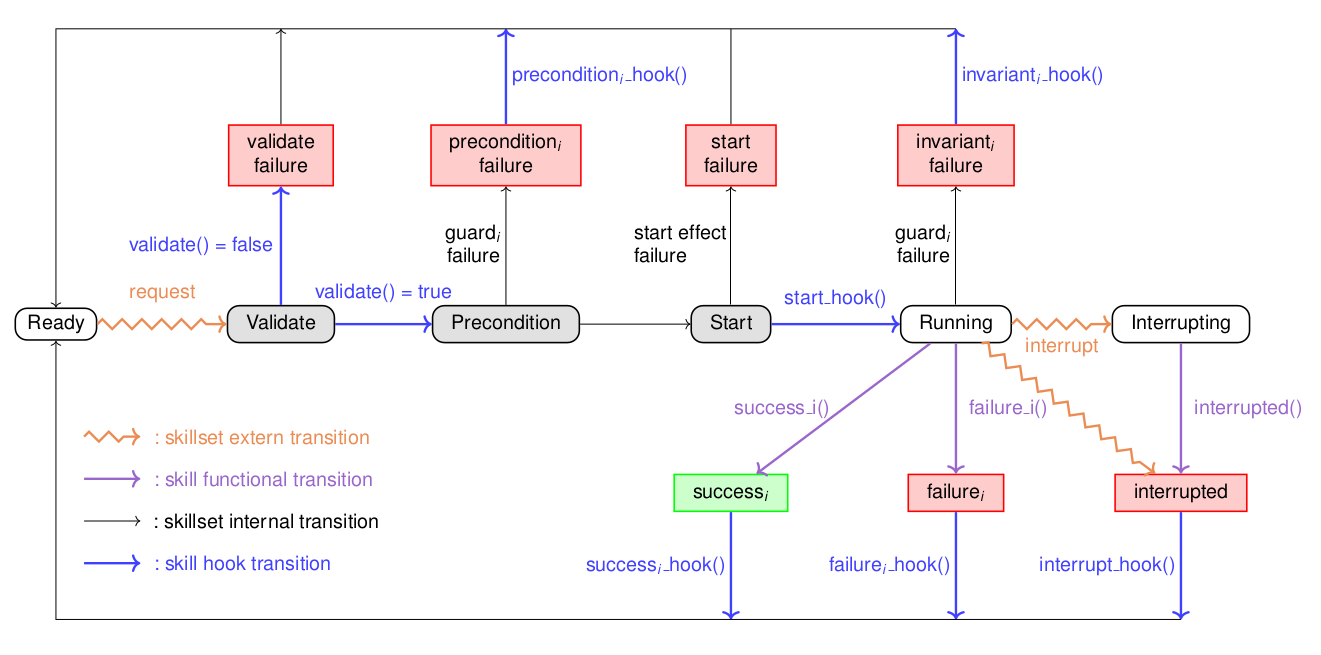
In this figure, the gray rounded rectangles represent the internal states of the skill state machine (precondition, validate, start). The white rounded rectangles represent the observable internal states of the skill. The rectangles represent the output states (red for failures, and green for success). The orange arrows represent requests from outside the skill: start or interrupt the skill. The blue arrows represent the different hook calls. The purple arrows represent the functional calls that interact with the skill. Note that to terminates a skill, the functional part must explicitly call the C++ function corresponding to the desired output state.
Moreover, each skill also has several hooks. Each hook is linked to a specific element of the complete behavior of the skill execution:
validate hook is used to define whether a skill request can start. This hook is only related to the functional elements of the skill and the inputs of the request (i.e. not the resources).
start hook is executed when the skill is started.
invariant hooks are executed if an invariant is violated. Each invariant has its own hook.
progress hook is executed when the skill is running at the period of the progress. This hook also aims to produce the outputs specified in the progress block (if any).
interrupt hook is executed when the skill is interrupted.
success hook is executed at the end of the execution of the skill if it terminates with a success. Each success state has its own hook.
failure hook is executed at the end of the execution of the skill if it terminates with a failure. Each failure state has its own hook.
Skill Input and Output
The skill inputs and outputs are defined with a name and a type, like the data are. Their types must have been defined. The next listing shows the inputs and the outputs of the takeoff skill.
1 skill takeoff {
2 input {
3 height: Float // [m] validate can fail if h>h_geo_fence
4 speed : Float // [m/s] maximum ascending velocity
5 }
6 output {
7 height: Float // [m] validate can fail if h>h_geo_fence
8 }
Skill Preconditions and Start
A skill can have several preconditions and only one start block. Each precondition is defined by its name, a guard, and can have effects. The start block simply has effects.
1 precondition {
2 has_authority: authority == Software
3 on_ground : flight_status == OnGround
4 motion_avail : motion == Available
5 battery_good : battery == Good
6 }
7 start motion -> Used
Skill Invariant
Each skill invariant is defined by a name, a guard and its effects applied when the invariant is violated.
1 invariant {
2 in_control {
3 guard motion == Used
4 }
5 has_authority {
6 guard authority == Software
7 effect motion -> Available
8 }
9 battery {
10 guard battery != Critical
11 effect motion -> Available
12 }
13 }
Skill Progress
While running, it may be useful to get information about the completion of the skill. This is achieved with the progress block, which has a period in seconds and outputs. During the execution, the skill will produce the specified outputs with the rate according to the period.
1 progress {
2 period 1.0
3 output height: Float
4 }
Skill Terminate
While running, a skill can finish its execution with an invariant failure or can terminate its execution by being interrupted, or can succeed or can fail. A skill can succeed (resp. fail) in different states, and each state has a specific hook and effects. The next listing shows the interrupt definition, the unique success definition, and the different failures of the takeoff skill. Sometimes a skill cannot be interrupted instantaneously. The purpose of the parameter interrupting is to specify the fact that the interrupt is instantaneous (set to false) or can take time (set to true). In this case, while receiving the interrupt, the skill switches to the ‘interrupting’ state and waits that the functional layer indicates the interrupt completion (interrupted). The postconditions are properties that represent the normal behavior of the skill. A postcondition can also be seen as part of the specification for the functional layer. The evaluation of the postconditions is returned at the end of the skill execution. Thus, if one fails, then the functional layer can react properly.
1 interrupt {
2 interrupting true
3 effect motion -> Available
4 }
5 success at_altitude {
6 effect motion -> Available
7 postcondition flight_status == InAir
8 }
9 failure {
10 grounded {
11 effect motion -> Available
12 postcondition flight_status == OnGround
13 }
14 emergency {
15 effect motion -> Available
16 postcondition flight_status == InAir
17 }
18 }